When starting out as a C# developer, you may be wondering how you can learn C# best practices so that you can write readable and efficient code. C# best practices are guidelines to ensure that you are doing the right thing and are just as important as the fundamental principles of coding in C#.
This article will guide you through some of the C# best practices that will help you get off to a good start in the web development industry. It also includes an overview of C# concepts, common challenges, and learning options that you can take advantage of.
What Is C#?
C# is a modern, object-oriented, general-purpose programming language mainly used on the Windows .Net platform, but it can be applied to any open-source platform. Although C# is a relatively new language, it is known for being reliable. It can be used to build secure, robust, and complex applications, including desktop apps, web apps, and web services.
It can also be used to create Microsoft applications that can be implemented on a large scale for game development in the game engine Unity. C# programming comprises static typing, strong typing, lexically scoped, imperative, declarative, functional, generic, object-oriented, and component-oriented programming.
9 Concepts You Need to Understand for C# Best Practices
In this section, we’ll go over some of the most important C# concepts that you need to understand before you can properly grasp the best practices and apply them.
- Attributes. Attributes are used to pass information or metadata on the behavior of elements within a program. This could be in the form of methods, classes, assemblies, properties, types, and structures. There are two types of attributes: predefined attributes and custom attributes.
- Iterator. An iterator is a block of code that is used in arrays or collections to retrieve individual elements. Iterators allow consumers of a container class to navigate the collections more easily.
- Variable. A variable is a container that is used to store different data types. In C#, each variable must have a type that will be used to determine the size and layout of the variable’s memory.
- VAR. In programming, you usually have to confirm local variables while stating their types. However, this is not essential with C#, as the program allows you to use a “var” type variable. “Var” means that you don’t have to create a new class for the result.
- Syntax. In C# programming, the syntax is the rules that define the language — the structure of the symbols, the punctuation, and words. Syntax is what gives programming languages life and makes it possible to understand them. Without syntax, the language would be useless and unreadable.
- Conventions. Conventions refer to the process of defining the names of variables, methods, and classes. Conventions are important not only for demonstrating C# best practices, but also for ensuring consistency, readability, and efficiency in a program, thereby increasing the quality of the code.
- Interfaces. An interface is a code structure that defines a contractual relationship between an object and its user, and may also define implemented static methods. It contains a set of related functionalities implemented by a non-abstract class.
- Constants. Constants are fixed values that do not change throughout the lifetime of a program, regardless of any execution process the program goes through. There are two types of constants in C# programming: runtime constants and compile-time constants.
- Object-Oriented Programming (OOP). OOP is a programming structure that allows a program to be organized around objects rather than action and logic, as other structures are. It is widely used in programming languages such as C#, Java, Python, C++, and Lisp.
5 Common Challenges That C# Guidelines Can Address
As a beginner, you can expect to face some difficulties while working on programs using C#. Below are some of the common issues that C# newcomers frequently face, but they can all be overcome if you adhere to the program’s best practices. By noting these potential problems and following C# guidelines, you will be taking an important step toward avoiding them.
Using a Reference Like a Value
In C#, the programmer who writes the object is the only one who can decide on the state of the values. That is, they can determine if the values assigned to variables are simply values or if they are references to existing objects. This is one of the most common mistakes new C# programmers make.
Using “Var” Needlessly
“Var” is very useful when it comes to dealing with unknown data types or an unexpected return of data. However, it is bad practice to overuse it. It is easy to keep using “var” in an attempt to save time, but it will negatively affect your code readability and will make it difficult for other programmers to use or maintain the code you’ve written.
Memory Leaks
It is normal to have memory or resource leaks in a program, which is why C# has a built-in method to dispose of these objects that are no longer in use. It is designed so that you only have to set or call an object in order to dispose of it. This helps to prevent memory leaks.
Using Iterative Statements to Manipulate Collections
Language-Integrated Query (LINQ) is used to manipulate and query collections, making it almost unnecessary for you to use iterative statements. So, if you get to the point of using iterative statements in your code, you have missed your chance to use LINQ.
Using Public Class Variables Instead of Properties
You should avoid using public class variables instead of properties because you can control who sets a property with object-oriented programming properties, but you lose that control if you use public class variables.
Top 10 C# Best Practices and Guidelines
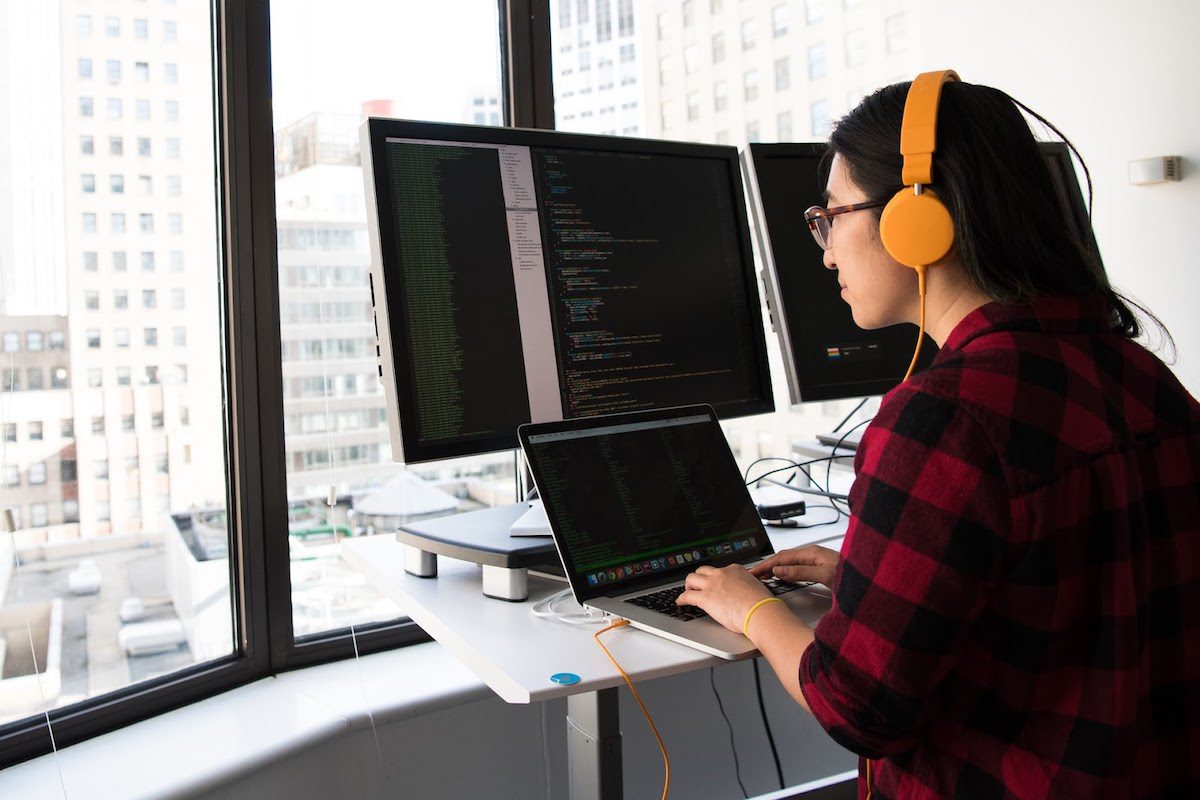
Best practices exist to help you write readable, efficient, and maintainable code that can be understood by other developers. By following the guidelines, you will be gaining the knowledge of experienced C# developers and will be able to avoid making some mistakes. Below are a few C# best practices that will help you get started on the right foot.
Use the Right Naming Conventions
Naming conventions show consistency in your code, so it’s important to use the right ones. C# is a case-sensitive programming language, with three naming conventions that are generally used: camel case convention, pascal case convention, and Hungarian case convention.
Pascal case convention is when the first character of all words is uppercase and the others are lowercase, for example, PascalCaseConvention. Camel case convention is when the first character of all words is uppercase except the first word, as in, camelCaseConvention.
Hungarian notation is not common, but it’s when the data type as a prefix is used to define the variable. Instead, it is best to use camel case when declaring variables and pascal when declaring properties. If all variables and method names are meaningful, it will increase the code’s readability.
Choose Between Value Types and Reference Types
When working on a C# program, you need to decide what type you want to use: value or reference types. Value types are commonly used when storing data, while reference types are used to create an instance of your type when defining the behavior. Reference types are polymorphic, while value types are great for memory use.
Value type variables are used to keep the original copy of a variable and to protect it from any unexpected changes, while reference types change the variable completely.
Use Properties Instead of Public Variables
Using properties instead of public variables keeps your code contained in an OOP environment and makes data validation easier. With properties, you can utilize getters and setters to prevent the user from directly accessing the member variables. If you explicitly restrict setting the values, you will be protecting your data from accidental changes.
Don’t Reinvent the Wheel
It is possible to create your entire coding library from scratch, but it likely isn’t the best option. You can simply use a tested and certified C# coding library. There are several libraries out there that will be useful to you as a beginning and will help you to prevent errors.
Establish Code Conventions
The minute you begin to work on C# projects, you will inevitably have a code style convention. This is because you will have already decided what format indentation to use, your preferred syntax, and the use of “var.” It is good to establish a code style because it ensures consistency and readability.
The best option is to automate your decisions and use a compiler or formatting tool to ensure that the decisions are enforced.
Use Prefix Interfaces with Letter ‘i’
The main reason that developers use the prefix “i” is that this piece of code is commonly used by C# developers, so if you use it, other developers will be able to read your code. Another reason to prefix interfaces with “i” is that the solution explorer in Visual Studio won’t have to distinguish between classes and interfaces.
So, the letter “i” is the best way to determine whether a file represents a class or an interface.
Use Nullable Data Types
C# allows you to store as null integer values, double, or boolean variables in certain situations. All you have to do is use the modifier “?” immediately after the type. This is a good option because the standard declaration doesn’t typically allow the use of null stored as a value. This will mostly come up when used with boolean properties.
Runtime Constants are the Preferred Constants
Runtime constants, denoted by the keyword “readonly,” are analyzed while the program is running. Compile-time constants are static values that are evaluated during compilation and are represented with the keyword “const.” Their value will not change each time the source code is executed. Because compile-time constants cannot be changed, they must be initialized when they are declared.
On the other hand, runtime constants can be altered at any point during the initialization process and can be set in the constructor. As a result, it is best to use runtime constants when writing reliable code and compile-time constants if you’re in a hurry.
Use Conditional Attributes
It’s a good idea to use the #if or #endif block to perform specific actions for the debug version of your code. However, there are some disadvantages, and you could end up with a bug in your code. This is where conditional attributes come into play.

"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Conditional attributes can help you avoid the pitfalls of #if and #endif statements. You can use these attributes by putting your code in a method and setting the conditional attribute to the DEBUG string. The method will be called when your application is in debug mode but not in all cases.
Only Catch Exceptions that You can Handle
Using the generic exception class to catch any exception will result in a lousy application with poor system performance. You should only catch what you expect and order it in accordance. If you want, you can add the generic exception at the end to catch any other unknown exceptions. This will make it easy for you to handle any potential problem.
How to Learn C# Best Practices
You can learn C# best practices by taking an online C# class, joining a coding bootcamp, reading a book, or going through relevant articles. These options are available to you regardless of your experience level and most can be completed online and at your own time. Some are even free.
If you’re a budding software developer, learning C# correctly is important. Having a strong educational resource helps you to avoid making mistakes that could be costly or have a lasting effect on your work or the work of the entire team.
Can a Bootcamp Help You Learn C# Best Practices?
Joining a coding bootcamp is a good way to learn C# best practices. Most C# coding bootcamps teach you relevant knowledge and assist you in developing critical skills that will help you transition into or start a career in technology. They will cover all you need to know about C#, including the fundamental principles and best practices that will help you start a career in web development.
Best Courses and Training Programs to Learn C# Best Practices
Provider | Course | Price |
---|---|---|
Coursera | C# Programming for Unity Game Development Specialization | Free |
Eduonix | Learn C Sharp Programming From Scratch | Free |
LinkedIn Learning | C# Best Practices for Developers | $29 |
Pluralsight | C# Development Best Practices | Free |
Udemy | Unit Testing for C# Developers | $84.99 |
Should You Learn C# Best Practices?
You should learn C# best practices, especially if you are new to the field. These are important guidelines that will help you write in a consistent coding style and format. If you stick to C# best practices, you will be able to write programs that will advance your career.
C# Best Practices and Guidelines FAQ
Some of the best coding practices in C# include using the appropriate case when naming your class and methods, and not using an underscore when naming identifiers. You should also always prefix an interface with the letter “i”, use conditional attributes when necessary, and use predefined data types over system data types.
C# naming conventions are part of the best practices for C# app development. They are the accepted standard for naming elements such as variables, methods, classes, and arrays.
The naming rules for C# are, only use pascal case when naming classes and methods. Camel case should be used for variables and method parameters. Avoid using Hungarian case convention to name variables (it’s outdated) and don’t use variable names that resemble keywords.
C# can be used to create any type of program, including complex applications for desktop and web, mobile apps, cloud-based services, enterprise software, and web services. It can also be used for Microsoft app development and Unity game development.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.