You can use the git clean command to remove untracked files. The -fd command removes untracked directories and the git clean -fx command removes ignored and non-ignored files. You can remove untracked files using a .gitignore file.
There are two types of files in a Git repository: tracked and untracked files. You may encounter a scenario where you need to remove untracked files from a Git repository.
Should we remove untracked files? We may have to do so if:
- Our working (local) directory cluttered by unused files
- You pointed Git to a folder you didn’t want to
- There are leftover files from other merges, or you want to remove certain files.
Git Removed Untracked Files
You can handle untracked files from a Git branch using these methods:
- A .gitignore file, which ignores the files and directories in a repository
- The git clean -fx command, which removes untracked and tracked files
- The git clean -fd command, which removes untracked files and directories
In this guide, we’re going to discuss how to remove untracked files with Git. We’ll refer to a few examples so you can get started quickly.
Difference Between Tracked vs. Untracked Files
In your working or local directory your files are either tracked or untracked. Tracked means those files added and committed in a previous snapshot and that Git is aware, tracking them for changes.
Untracked files are the opposite, those files were not in the previous commit and have not been staged to be committed. You have the option of either stage them and commit them to your repository, or remove them!
If we do git status right after modifying/added files it would show us the list of untracked files and files that are tracked.
Remove Untracked Files Git Option 1: .gitignore
The first option is to ignore such files. You could be working on a C++ project that during build you might get files generated you don’t want available.
For instance, you may have a .env file with all your environment variables and database, API, access keys. You wouldn’t want that info out there in the wild either right? This is where .gitignore files come in to play.
Any files that are present in a .gitignore file will not be part of the untracked/tracked Git flow. They’ll be removed from it. So Git will ignore them and not track them nor complain they are not tracked.
First, let’s create a .gitignore file in root. And then we’ll specify the relative path of the location. So if let’s say we want to hide the node_modules and config.env file we just add them as such:
node_modules config.env
Understanding Gitignore is essential as your project grows so you must be aware which folders or files need to be added there. This is because you don’t want them in the Git workflow as untracked to then stage them by mistake and have Git tracking such sensitive files for everyone to see your keys exposed!
Understanding Gitignore is essential as your project grows so you must be aware which folders or files need to be added there. This is because you don’t want them in the Git workflow as untracked to stage them by mistake. This would cause Git to start tracking files with information that should not be shared in a repository.
Remove Untracked Files Git Option 2: git clean
The next option we have to remove the files is to use the git clean command. The git clean command deletes untracked files from a repository.
The git clean command starts from your current working directory inside your working tree. Your working tree is the branch you are viewing.
This command would be useful in case, for instance, you accidentally add a folder with high school pictures into a repository instead of another folder.
Git clean takes a couple of options. Let’s take a look at the syntax for this command:
git clean [-d] [-f] [-i] [-n] [-q] [-e <pattern>] [-x | -X] [--] <path>…
At this point it is worth mentioning that Git clean deletes your file in a hard system way. This means you cannot undo your changes. It is similar to the rm command in the terminal. But, if a file was tracked in a previous commit, we may be able to find an old version.
git clean -d -n
If we don’t specify a path we often want to include the -d option to have Git look into untracked directories.
We should include the -n dry run option first. This instructs Git to warn us what will be removed before deleting it. In other words, the -n flag performs a dry run of our clean function. Then, we can run git clean once we are sure our changes have been made.
Using this option will give us a safeguard against removing files that we are not ready to remove, or do not want to remove. Here’s how we end up with the git clean -d -n command.
Let’s look at this in action. We have our sillyPicture.jpg we don’t want to track and want to remove. Check what will happen:

git clean -f
In order to get Git clean to work, we may need to specify a force option. This option should only be used if you are sure that you want to remove the files you have selected from a repository.
Let’s go ahead and remove our sillyPicture.jpg with git clean -f or you can be more specific with git clean -f sillyPicture.jpg
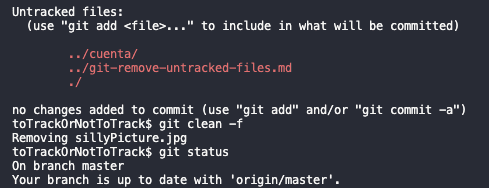
Here you might notice that here git status first pointed out there was an untracked file, then after we deleted with the -f option the file was then gone. C’est fini!
Although the -f option and Git clean is very powerful, it will not work with ignored files. This because it only works with files Git is aware of and are part of its version control system.

"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
git clean -x
This is not recommended unless you really know what you are doing. Yes you can remove ignored files and directories. To do this just use the -x option. So git clean -d -x -f will do that as easy as the blink of an eye. But don’t use it, just yet.
git clean -d -i
Recommended for beginners. As you’re getting started you might want to be careful of what options you pass in. We already showed you the dry run -n option. There’s also the -i option that will show you an interactive interface you can play with!
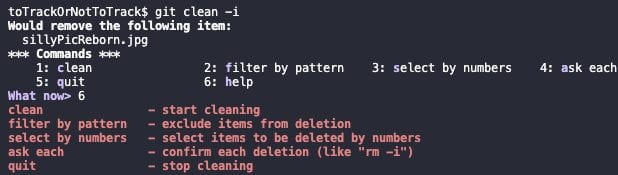
Conclusion
You can remove untracked files from a repository using either the .gitignore or the git clean command. The git clean command removes files recursively. This command starts in your current working directory.
To learn more about Git, read our How to Learn Git guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.