Over the last few years, React has established itself as a go-to library used for the development of user interfaces. This open-source JavaScript library is packed with resources to help developers create websites and applications quickly and efficiently. If you want to know how to learn React and leverage this resource to advance your coding career, you’ve come to the right place.
In this guide, we will discuss how you can learn React from scratch, the top resources that can help you along the way, and even the best way to learn React using free online resources. But first, we’ll discuss some React basics to help you understand the benefits of using this library. Let’s dive in.
What Is React?
React.js is an open-source JavaScript library. It is mainly used to create user interfaces and components for a web page. The library is maintained by Meta as well as a community of independent developers and companies.
With React, you can bundle up different coding building blocks that comprise the library to use them for building simple websites or applications. This allows you to reduce repetition and increase performance for whatever products you’re creating. According to the 2022 Developer Survey by Stack Overflow, React.js is among the top frameworks used by developers, topped only by Node.js.
How Long Does It Take to Learn React?
On average, it takes between one and six months to learn React. The exact time to master React depends on your software development experience and the time you are willing to dedicate to learning.
If you spend 10 hours a week studying consistently, you’ll learn React fast, in around a month. If you spend two hours per day learning React, you should be able to master the fundamentals within a few months.
These time frames do not take into account how long it takes to learn React if you have no programming experience. Because React is a JavaScript library, the first thing you’ll need to do is master the fundamentals of JavaScript. Then, you’ll be ready to start researching how to code using React.
Prerequisites for React: HTML, CSS, and JavaScript
As a prerequisite for learning React, you need to have knowledge of fundamental programming languages. This is because React is a library that is based on JavaScript. And before you start coding using React, you should not only learn JavaScript but also HTML and CSS.
While HTML and CSS are not required, the JSX syntax used by React is based on HTML. You’ll need to have a good knowledge of HTML to understand how it works, and a working knowledge of CSS will also help you implement the resources from the library more effectively.
Best Resources to Learn JavaScript
Below is our list of the best resources to learn JavaScript you can use to begin your coding journey. Some resources are available for free, while others are paid. Once you master the JavaScript coding basics, you’ll be able to use React to build websites and apps.
- The best JavaScript bootcamps are a great way to build a solid foundation using this language.
- The site freeCodeCamp.org provides you with real-world projects to help you learn JavaScript.
- Udemy’s Learn to Program in JavaScript is an excellent free course that will teach you the essentials.
- CodeAcademy, a top coding bootcamp, offers a free course to learn JavaScript.
- The JavaScript Basics by Mozilla can also help you learn the fundamentals.
How to Learn React: Piece by Piece
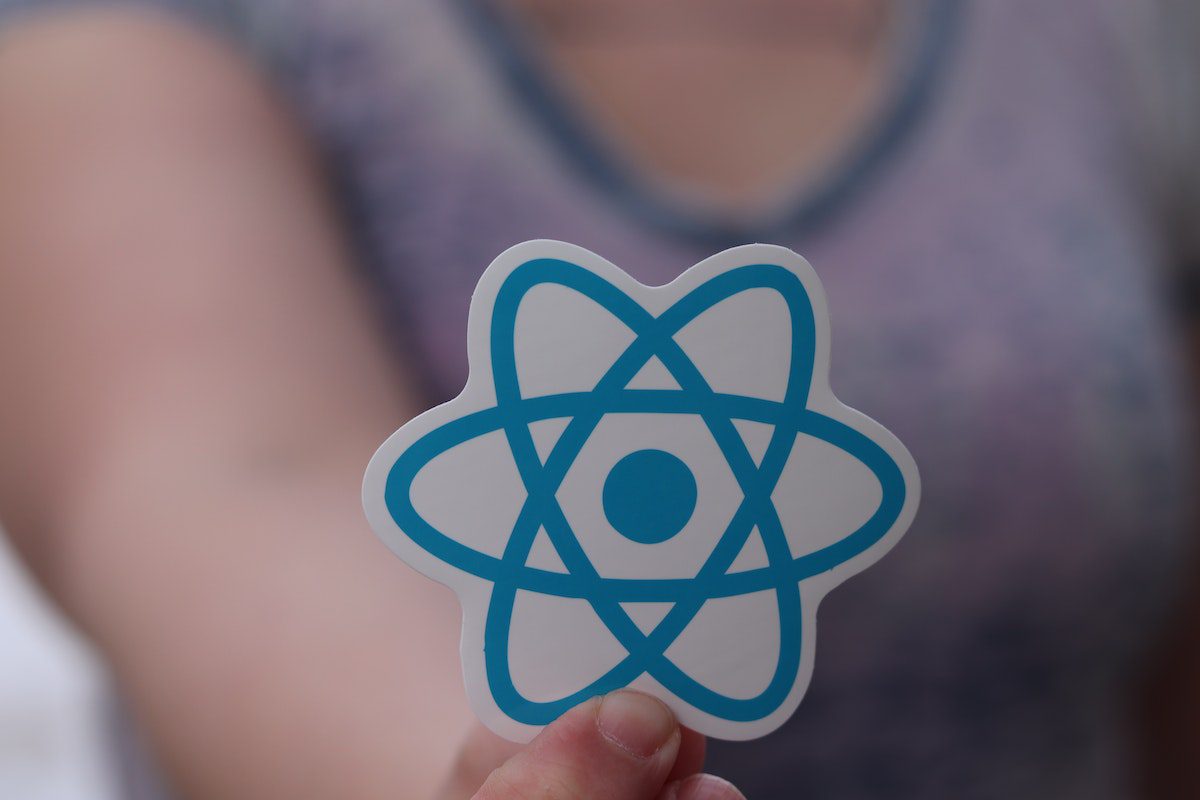
If you’re ready to take the leap and master this library, you might be wondering exactly how to learn React. In order to use React effectively, you’ll need to learn different skills that you can then combine. Keep reading to learn about the different React essentials and how you can master each one.
JSX
JSX, or JavaScript Expression, is a syntax extension for JavaScript written for use with React. The JSX technology allows you to describe how a user interface should appear on a website. You should start learning React basics by exploring how JSX works. Read about how you can use JSX to create elements in a user interface.
Here are a few topics you should explore to further your understanding of JSX:
- What is JSX?
- What is a JSX element?
- How to work with attributes
- Nested and outer JSX elements
- Rendering elements using JSX
- Rendering elements using the React DOM
- The Virtual DOM
React Router
Routing shows how users will move throughout your website. It’s a tool that links a URL and your application. Consider this example. You’re on your homepage, and you’ve clicked and navigated to your bio page. This is routing in action.
React Router allows us to build a single page web application without the page refreshing as the user navigates. Router uses a component structure to call components, which holds and displays the appropriate information.
Components
Components are the building blocks upon which all React JS-powered applications are built. All web pages that use React use components to declare each part of the site. For instance, a navigation bar may be a component, or an article on a web page may be a component. In turn, the article component could consist of a comment component or a text component.
Components allow you to develop user interfaces that are easier to maintain and load quickly. Here are the main topics you should master when it comes to React components:
- How to create a component class
- How to render a component
- How to create an instance of a component
- How do components interact
- How to use components in a render function
- How to reference components from another file
- Controlled and uncontrolled components
Lists
Lists are an important part of any website. Building lists is a great way to familiarize yourself with the React fundamentals. As you start to learn about React, you should spend time learning about how you can render lists of data on a web page.
States and Props
A state refers to how each object on a web page appears. Every component in a React app can have its own state. Props, on the other hand, are used to share code between components. To work with React, you need to have a good understanding of both states and props. Here are the main topics you should delve into.
- What is the React state?
- this keyword
- How to use props in React
- PropTypes
Webpack
Webpacks are bundles of files and codes, also known as assets. A React Webpack is a command-line tool you can use to create these bundles, which are large files that are essentially reusable packages that can be easily used in a web browser.
React Redux
Redux is a JavaScript library that makes it easier to manage the “state” of your application. Think of the state in terms of what you’ve learned about components at this point. The state of a component is like the props which are passed to a component, like a plain JavaScript object. This object contains information that influences the way that a component is rendered. In essence, Redux helps you manage the data you display and how your site responds to user actions.
Advanced React Concepts
We have only scratched the surface of React JS concepts. Because the library is so popular, there are frequent updates made available. These updates continue to add even more features to make React even more useful. These are some of the advanced React concepts you can move on to once you’ve mastered the basics.:
- Higher-order components
- React context
- React hooks
- React render prop components
- React icons
- React forms
- React Native
- Handling API requests using React
How to Learn React Online for Free
There are various resources you can use to learn React online, which are maintained and divulged by the massive developer community supporting the library. Keep reading to learn more about these resources so you can start learning React for free.
Best Online React Resources
Below is a list of the best resources to learn React online. These websites provide you with documentation and guides to orient you during your learning journey. Make sure to explore them and discover which ones are best suited to your learning style.
- Learn React Documentation. The official React documentation is one of the best sources of content when it comes to learning React. The React documentation is updated with every change to the library. This means that everything you read on the site should reflect the latest version of React.
- React Resources. React Resources is a directory of resources for people who work with React. You’ll find plenty of guides to assist you along the way, divided into categories such as podcasts, books, and talks.
- Getting Started with React. This guide by freeCodeCamp covers the fundamentals of React. You’ll learn to build a small project that retrieves data from an API by following this guide.
Best Free React Courses
Another great way to learn React is to follow along with free React courses online. The best courses to learn React are like a class you would attend in college, but you get to control the pace at which you go through the course. And, you can always rewind a video if you get stuck. You can also check the React Community course list to find alternatives.
- React Starter Kit. React Starter Kit is a free, five-part course that introduces you to React. This course is accompanied by code snippets that illustrate the concepts covered in the course.
- Codecademy: React 101. Codecademy has an online course covering React development. You’ll learn about JSX, lifecycle methods, hooks, and everything else you need to know to build a web application using React.
- Scrimba: Learn React for Free. Learn React for Free is an online course with 48 video tutorials. You’ll cover topics like ReactDOM and conditional rendering in depth.
Best React Tutorials
The best React tutorials on YouTube cater to any aspiring React developer. Besides React courses and coding challenges to help you practice, tutorials usually hone your React development skills with step-by-step instructions or processes. If you want to get better at front end development, learning the fundamental concepts of React through these tutorials will surely give you an advantage.
Tutorial | Creator | Length | Level |
---|---|---|---|
Advanced React Patterns, Performance, Environment, and Testing | codedamn | 58 minutes | Advanced |
Advanced React Tutorials | techsith | 33 videos | Advenced |
Full React Course 2020 – Learn Fundamentals, Hooks, Context API, React Router, Custom Hooks | freeCodeCamp.org | 10 hours | Intermediate |
Full React Native Project Tutorial for Beginners | Cryce Truly | 10 hours | Beginner |
Full Stack React & Firebase Tutorial – Build a social media app | freeCodeCamp.org | 12 hours | Intermediate |
Junior vs. Senior Code – How to Write Better Code as a Web Developer – React | Web Dev Simplified | 21 minutes | Intermediate |
Learn React JS – Full Course for Beginners – Tutorial 2019 | freeCodeCamp.org | 5 hours | Beginner |
React Advanced Concepts | Every React Developer Should Know | CodeBucks | 7 videos | Advanced |
React Crash Course for Beginners 2021 – Learn ReactJS from Scratch in this 100% Free Tutorial! | Academind | 4 hours | Beginner |
React JS Course for Beginners – 2021 Tutorial | freeCodeCamp.org | 7 hours | Beginner |
React JS Crash Course 2021 | Traversy Media | 1.5 hours | Beginner |
React JS – React Tutorial for Beginners | Programming with Mosh | 2.5 hours | Beginner |
React JS Tutorial for Beginners – Full Course in 12 Hours [2021] | Clever Programmer | 11.5 hours | Beginner |
React Tutorial for Beginners | developedbyed | 43 minutes | Beginner |
React Website Tutorial – Beginner React JS Project Fully Responsive | Brian Design | 1.5 hours | Beginner |

"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Advanced React Patterns, Performance, Environment, and Testing
Gain a deep understanding of advanced React concepts through this hour-long video and learn how to create stylish and functional websites using React, CSS, APIs, and more. The tutorial mainly focuses on compound components, an advanced React pattern to achieve flexible APIs, and reliable server connections. You will also learn how to test the performance of your React app to deal with issues encountered while building and debugging.
Advanced React Tutorials
Create dynamic user interfaces, optimal mobile applications, and web pages through this series of advanced React tutorials. The tutorials discuss React hooks and how to create your app without classes to easily reuse certain components for other functions without modifying others.
There is also a wide range of React programming concepts such as pure components, refs, TypeScript, and DOM. These topics are discussed thoroughly through several videos, which will help you to build better web or app interfaces.
Full React Course 2020 – Learn Fundamentals, Hooks, Context API, React Router, Custom Hooks
A 10-hour full React tutorial is available for free on YouTube by freeCodeCamp if you want to build your knowledge on essential concepts to advanced techniques in React. Through React hooks, CSS, and other elements, the goal is to help you master building a dynamic user interface for web and mobile platforms. This comprehensive tutorial also goes deep into React Router.
Full React Native Project Tutorial for Beginners
This free tutorial on React Native helps beginners learn how to build Android and iOS applications. Since this tutorial does not deal with single-page applications, it runs for 10 hours as it covers how to create functionalities like user login or password options. The last few sections are about achieving a responsive layout and how to optimize your application depending on the screen size of your device.
Full Stack React & Firebase Tutorial – Build a Social Media App
This 12-hour course is a comprehensive tutorial on building a social media app using React and Firebase, which is a reliable platform to develop applications smoothly. This tutorial is project-focused and will teach you how to enable essential social media app functions such as registration and uploading your image.
You will also learn how to add user details and how to like and unlike a post. Once you have completed the entire process of building the app, the last part of the tutorial provides a step-by-step guide on deploying your application to Firebase.
Junior vs. Senior Code – How to Write Better Code as a Web Developer – React
This short tutorial video delves into the contrast between a junior developer and a senior developer to improve your React coding skills. You will gain insights into how senior full stack developers think and grow more comfortable with state and effect in React. More importantly, this tutorial conditions your mind to think like a programmer, so you will have a well-rounded understanding of React and be able to plan out your future projects better.
Learn React JS – Full Course for Beginners – Tutorial 2019
This in-depth five-hour React tutorial by freeCodeCamp starts with an introduction to React and the philosophy behind it. As you learn more about the core concepts of React JS, the tutorial expects you to build your own dynamic web application. There is an in-depth discussion on how to design your React application together with CSS classes and how to generate or brainstorm more projects and ideas to practice your skills.
React Advanced Concepts | Every React Developer Should Know
This series of tutorials is ideal for any aspiring React developer wanting to master advanced React concepts. It deals with topics such as pure and higher-order components, as well as refs and forwarding, which enable components to connect with one another. There is also a dedicated tutorial for error boundaries, which is essential to identifying and addressing errors in components to prevent running into problems.
React Crash Course for Beginners 2021 – Learn ReactJS from Scratch in this 100% Free Tutorial!
Maximilian Schwarzmüller offers a crash course for beginners that focuses on building an application with React JS. The tutorial emphasizes the importance of components because this will determine what the application looks like on-screen.
If you are an aspiring front end developer, you will gain a basic understanding of styling using CSS classes through this tutorial. For example, you will learn how to prepare your application for the web and ultimately deploy it for use.
React JS Course for Beginners – 2021 Tutorial
Another tutorial by freeCodeCamp, this video is available for free and runs for more than seven hours. This tutorial is ideal if you want to have a strong understanding of React and full stack web development.
The tutorial drills deep into components, props, and states. There are also sections on how to use React with Bootstrap, which is helpful for mobile app development to achieve a dynamic interface regardless of screen size.
React JS Crash Course 2021
React JS Crash Course 2021 is another free tutorial on YouTube where you will learn about components, hooks, state, and props in under two hours. The goal is to help you get started by building a task tracker app, so this tutorial will also teach you how to utilize JSX and JSON servers. Finally, it also focuses on APIs as the task tracker app will need to connect with a server for adding and deleting functionality.
React JS – React Tutorial for Beginners
Learn the core concepts of React JS through this two-and-a-half-hour tutorial by Mosh Hamedani. The first part introduces you to React and how to set up a development environment, which you will need to make sure that there are no interruptions while working on your project.
The tutorial focuses on basic concepts such as expressions, attributes, classes, and lists. You will also learn how to debug React applications when you run into problems, which will strengthen your resolution skills.
React JS Tutorial for Beginners – Full Course in 12 Hours [2021]
Learn React and JavaScript through this 12-hour beginner tutorial where you break down and study its different elements through popular media applications. This tutorial focuses on Netflix, Spotify, Slack, and TikTok and covers what makes each one of these applications unique. This approach will help you create your own website or application and improve your web development skills by understanding popular apps.
React Tutorial for Beginners
If you are looking for a shorter tutorial, this 43-minute video for beginners covers the basics of building an application through React JS. The tutorial starts with instructions on installing React, then provides an in-depth discussion of components, and mainly focuses on writing code with React hook. It also teaches you how to pass props, which is essential when sending data from one component to another and is required for any application to work optimally.
React Website Tutorial – Beginner React JS Project Fully Responsive
Learn more about full stack development with React JS through this tutorial on creating a website from scratch. This tutorial will guide you on how to get started with website development using React, highlighting the importance of components and how to create pages. There is also a section discussing how to replace a video background with an image using React. Additionally, it covers how to do an overall polishing of your site or application.
The Best Way to Learn React
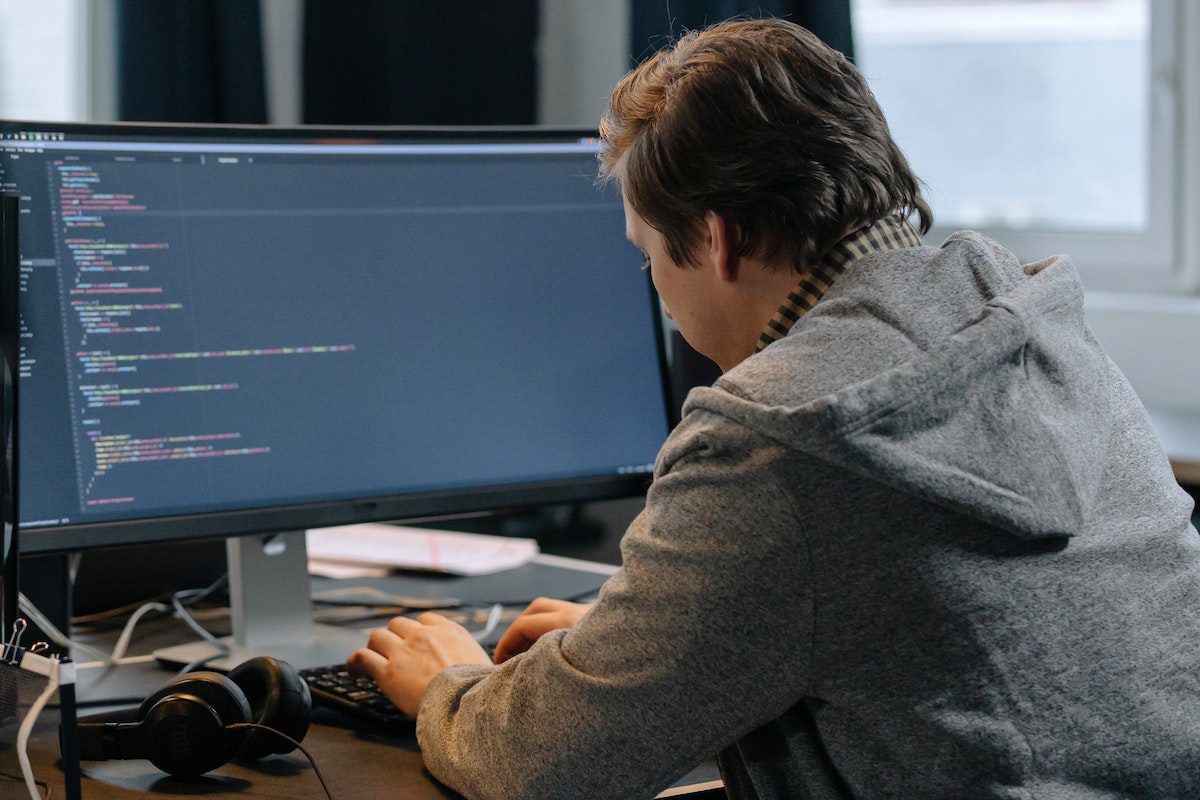
The best way to learn React is to choose resources according to your learning style, career goals, and current level of programming prowess. However, regardless of the path you choose, there are a few things you can do to ensure you will succeed. Keep reading to see a roadmap to learn React that will hopefully support you in your learning journey.
Get Set Up
Your first step in learning React should be to set up your work environment. Below are some of the tools you will need to install on your computer to start working with React:
- Install NPM. NPM (Node Package Manager) is a site that allows you to install libraries into your React project. You’ll need to use NPM to access external libraries and to install React itself.
- Choose an IDE. IDEs are the development environments in which developers build applications. For React developers, Visual Studio Code (VS Code) is a popular choice, alongside Atom.
- Use Prettier. Your React code can become messy quickly. That’s why it is handy to have a tool like Prettier at hand, which can format your code in your development environment. Prettier has a custom React feature you can use to format your React code.
- Try out create-react-app. create-react-app is a boilerplate that gives you everything you need to start a new React app. Many tutorials recommend that you start from the create-react-app project because all the basics are configured.
Practice Your React Skills
Building projects is an excellent way to sharpen your React skills. There is only so much you can learn through passively watching YouTube tutorials, so make sure to get your hands dirty and put everything you learn into practice through projects that will help you understand how React works and the advantages it offers.
In addition to completing projects, you can also participate in coding challenges to help you get some practical experience. A challenge such as 100 Days of Code is a great way to make a commitment to practicing and also gives you a chance to connect with a community of students, which can prove invaluable during your learning journey.
Join a React Community and Get Feedback
Developer communities are places where you can go to talk about code, share your ideas, collaborate on projects, and receive feedback. They also give you a chance to network with peers, discuss the challenges you run into, and get support from people who understand the path you’re on. Communities such as StackOverflow or Dev.to are a great place to start.
Should I Learn React?
Yes, you should learn React if you’re interested in becoming a developer and want to master a tool that can help you write better websites and apps in a shorter time. If you’re wondering how to learn React, rest assured that there are many resources you can use to master the essentials and start building simple projects, as we’ve seen in this guide.
Because of its popularity and ease of use, React libraries are constantly updated and continue to grow, filling up with newer resources you can leverage to succeed in the tech world. If you are interested in building efficient products for your clients, learning React will be a worthwhile investment.
How to Learn React FAQ
React is not hard to learn, but before you start looking at guides and tutorials, you need to have a solid understanding of JavaScript. Knowledge of HTML and CSS can also make your React learning journey easier.
Some good books on React that can support you in your learning journey include The Road to Learn React by Robin Wieruch, Learning React by Alex Banks and Eve Porcello, and Pure React by Dave Ceddia. Each book approaches React from a different perspective, so make sure to check the contents and read some reviews to ensure they are the right fit for you.
The answer to whether you should learn React, Angular, or Vue depends on the type of project you will be working on. Vue is generally regarded as easier to learn, but both React and Angular are more popular. Study your market and learn which tool is more commonly used to help you decide which of these tools you should master first.
Yes, you can learn React with TypeScript. An advantage of taking this path is that TypeScript can reduce the chance of errors in your code, and offers an easier way to track changes to specific data segments. However, it is not necessary to master TypeScript to learn React, what’s essential is to have a solid understanding of JavaScript.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.