As you search the web, you’ll find many articles about React.js, also referred to simply as React. This post is written not as a step by step guide to creating your first React application, but instead as a strategic roadmap to follow that will make learning React easier.
If you want to learn how to create your first React app, I’ve listed some great resources within this post. This library can be a bit overwhelming to learn at first, so I recommend that you read this post and follow the roadmap provided. Keep this handy as you go through your React training and use it as a checklist.
What is React.js?
Before we dive into this discussion, let’s define what React.js is and what it is not. React.js is not a language or a framework; it’s a library created by Facebook and maintained by both the company and the open-source community–and a library that makes writing JavaScript a hell of a lot easier. Its main purpose is to create fast, scalable, and simple web applications. React uses a component structure which allows for easy reusability of code. It also allows you to create content that has the ability to change without reloading the entire page via an in-memory structure.
If you’re a person who finds JavaScript structure a bit overwhelming, React.js will become your best friend if you decide to learn it.
Is it the Right Time for You to Learn React?
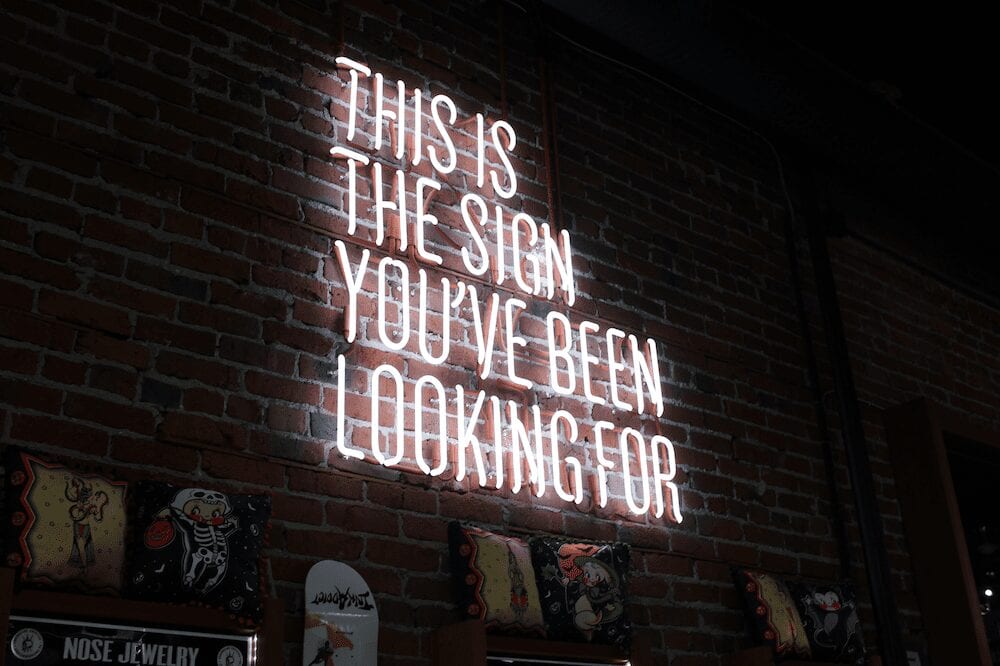
A question that I see circulating around the internet lately is, should developers, especially new developers, become fully proficient in JavaScript before deciding to learn React. My response is, if JavaScript is your first language, I would spend some extra time before moving on to this library. However, you don’t need to be fully proficient in JavaScript before learning React. React will continue to increase your JavaScript skills. This is not to say that you should study JavaScript for one week and expect to be ready.
Here are 11 topics you should know about JavaScript before moving on to React:
- Basic Syntax (This includes: Arrays, Variables, Parameters, Arguments, and Methods)
- Object Literals and Template Strings
- ES6 Classes
- Block Scope
- Arrow Functions
- Map and Filtering
- The Difference Between Const and Let
- Destructuring
- Promise Objects and How to Use Them with Async and Await.
- Imports and Exports of Modules
- Basic Understanding of Npm
Another piece of advice: as you move on to React, you’ll want to continue improving your vanilla JavaScript skills through code challenges. Sharpening your logic is key to success. Keep in mind that this tactic will not only help you with React and JavaScript but also help you become a stronger developer overall.
Where to Start on Your React Journey–A Roadmap
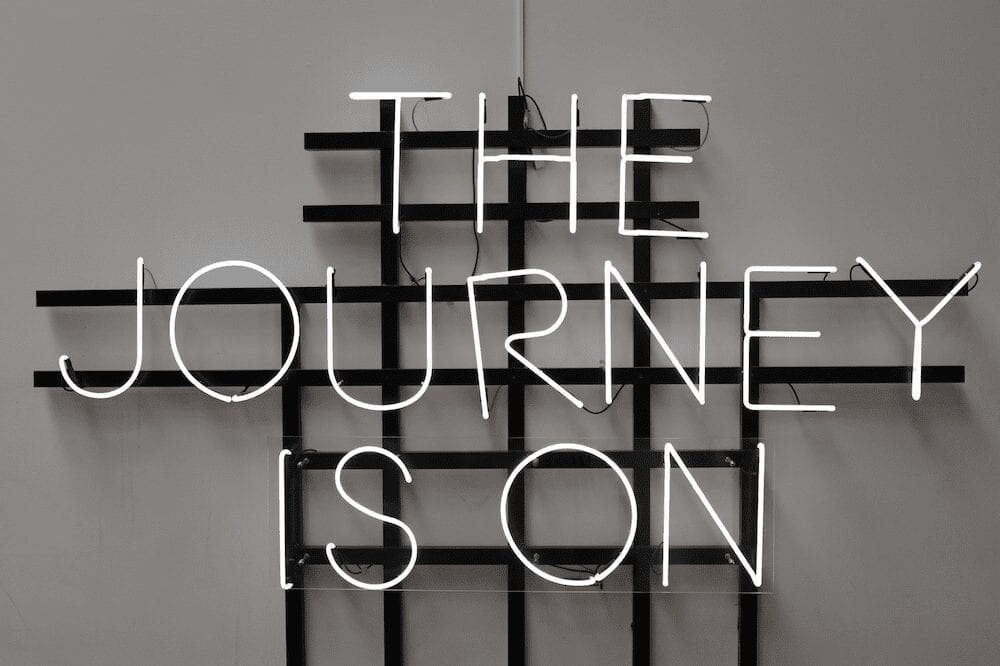
So you’ve learned the above, you’ve confidently passed a few somewhat intimidating code challenges, and you now feel ready for React.js. Here is a quick road map to guide you along your React journey.
First Stop: The Basics
Let’s build out the basics! The best way to learn is to dive in headfirst. Learn the basics of creating a React app. Sources I recommend are freeCodeCamp, Scrimba, and Reactjs.org. Reactjs.org can be a little overwhelming for beginners, but it’s a great resource for all things React.js, so it’s worth getting used to.
Topics to cover if you want to ask the Google gods:
- React JSX Syntax
- React Components and Composition
- React Props
- React State Management
As you advance in your training you will want to learn:
- React Hooks
- React Higher-Order Components
- React Render Prop Components
- React Context
With the above in mind, a great project to begin with is to create a simple to-do app. Here’s a great resource to begin.
Why a to-do app? If you are new to development, soon you’ll realize how the functionality of a to-do app correlates with other projects you’ll create in the future. When I began my React journey, a good developer friend explained it to me well: “You’ll soon see that most things are a to-do app”. You’ll understand this statement soon–just trust the process.
A piece of personal advice I’d like to share is to avoid purchasing beginner courses from paid sites because there are tons of free resources to get you started. I know plenty of developers, including myself, who’ve purchased many beginner courses and still have not completed half of them. It felt like collecting and sharing Pokemon cards, please avoid trying to catch ‘em all. This in itself can become an overwhelming and expensive task of trying to find the right courses to buy after doing extensive research. Let’s try not to exhaust ourselves before we even begin.
Second Stop: Routing
Let’s introduce Routing! It’s time to build out a more dynamic application. Routing, in plain English, shows how users will move throughout your website. It’s a tool that links together a URL and your application. A visual example of this: you’re on your homepage, and you’ve just clicked and successfully navigated to your bio page–this is a result of routing.
React Router allows us to build a single page web application without the page refreshing as the user navigates. React Router uses a component structure to call components, which holds and displays the appropriate information.
Is this concept still a bit unclear? Check out this introduction to React Router.
According to the React documentation: “React Router keeps your UI in sync with the URL. It has a simple API with powerful features like lazy code loading, dynamic route matching, and location transition handling built right in.”
To the new developers in the room, if the terms API, UI, and URL are unfamiliar to you, ask the Google gods. Get used to doing so: Google is now your career coach.
Third Stop: Redux
Let’s learn Redux! It’s a good idea to become very familiar with step two before moving on to Redux. What is Redux? It’s a JavaScript library that makes it easier to manage the “state” of your application. Think of state in terms of what you’ve learned about components at this point. The state of a component is like the props which are passed to a component–like a plain JavaScript object containing information that influences the way that a component is rendered.
Did that confuse you? Another way of looking at Redux is that it helps you manage the data you display and how you respond to user actions. Redux makes state management much simpler; however, there is a bit of a learning curve when getting started.
The Advice You Should Follow While Learning React, or Anything New
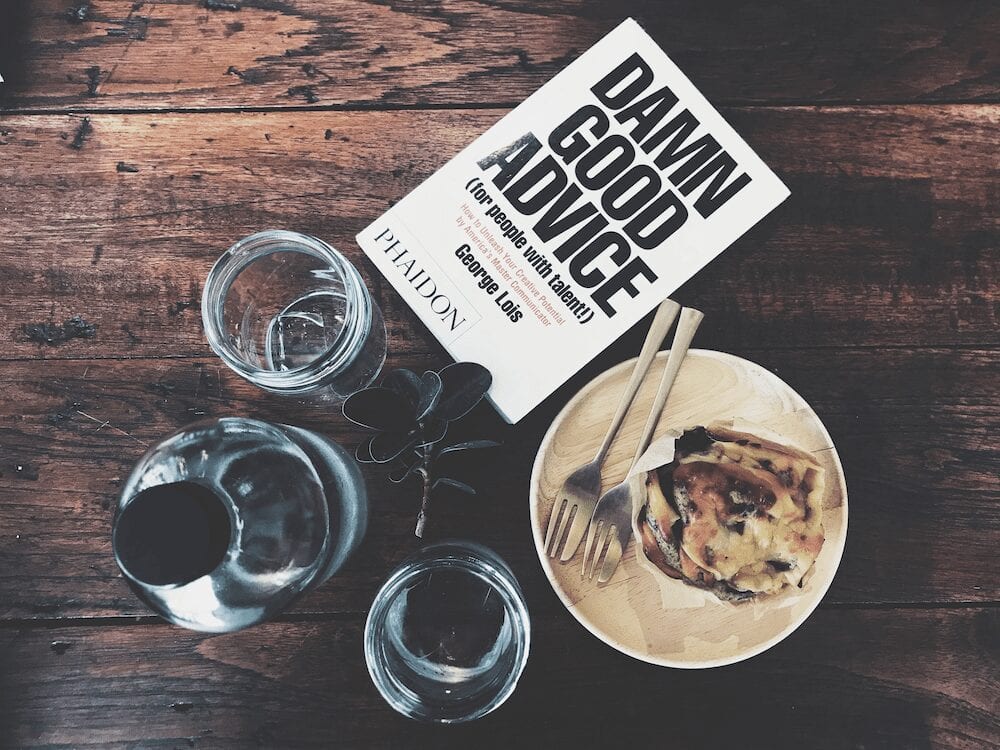
At this point, I’ve given you quite a bit of advice in a condensed fashion. Reach out to me, if any of the above isn’t clear or simply overwhelms you. I’ll try to end this by not stating the obvious which most blog posts state: “repetition is key!”
This is true, but other ways I recommend increasing your React.js skills are:
- Through blogging about what you’ve learned and any new topic on React that interest you. If you can explain what you’ve learned, then you are ready to move to a new topic.
- Show off your work via social media and let your followers praise and bestow constructive criticism. This not only allows you to see things through other’s perspective but also keeps you accountable for the quality of your work.
- Another option is to search for React-related events in your area. As developers, we devote a lot of time in front of our computer screens. Get out, learn, and make some new dev friends! Don’t be a hermit.
My final piece of advice.

"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
While picking projects, make sure to increase the complexity as you continue to build. Let me add that following tutorials are a great way to start building, but you must get confident enough to build complex applications from scratch. That’s right, map out your personal project and build it yourself.
Good luck! You can do it! I believe in you!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.