Learning the essential tools of a web application developer can be a constant journey. There are many coding languages and frameworks that you can use to see your project through. Of these many frameworks, some are more popular than others because they can be used to create better apps. One of the best frameworks for creating high-quality apps is Angular.
Angular was designed to make front end and backend applications more manageable. It uses static typing, which many programmers prefer because it helps catch coding errors. In this article, we’ll highlight some Angular best practices and common challenges that you may face while working with the framework.
What Is Angular?
Angular is a free, open-source framework that’s used to build single-page client applications with the programming language HTML and TypeScript. It was developed by the Angular Team at Google in 2016. Angular can be used to build native apps for mobile devices and desktop computers and is a particularly good platform to use when building large enterprise apps.
Angular is a rewrite of AngularJS, an open-source JavaScript framework also developed by Google several years prior. AngularJS was designed to develop front end applications and was popular in part because it supports static HTML pages. However, it had to be rewritten due to drawbacks, hence, the launch of the new Angular version.
10 Concepts You Need to Understand for Angular Best Practices
There are certain key terms and concepts that you need to understand if you want to use Angular. Studying the terms below will make it easier for you to understand and navigate the framework.
- Modular Angular architecture. One of the most important aspects of Angular architecture is the use of modules. An Angular app is made up of modules that are structured in a way that will allow you to scale up your application as it grows.
- One-way dataflow and immutability. This dataflow process, also known as unidirectional data, ensures that data moves from top to bottom and that any changes are circulated throughout the system. This means the app can avoid problems like performance overhead.
- Attribute and structural directives. Directives are extensions of HTML through custom elements. Attribute directives alter the appearance or behavior of DOM elements. Structural directives are used to change the DOM layout by shaping or reshaping HTML.
- Application structure and best practices. To use Angular effectively, you must first understand its structure. This includes understanding the requirements of an Angular app, and how everything fits together on a practical level and builds from the module root..
- Template binding syntax. Template binding syntax acts as the facilitator between static HTML and the JavaScript framework. It makes it easy to turn a static page into an interactive page. Some scenarios in which you would use template binding syntax are for property binding, events, interpolation, and two-way binding.
- Feature modules and routing. Feature modules can be used to organize business requirements and prevent code pollution. There are five different types of feature modules. Routing is the path the data takes within your app as it’s being transferred.
- Forms and validation (reactive forms and validators). Forms are necessary when working on front end development if you want to create a strong application performance. The best way to construct effective forms in Angular is to use reactive forms to improve your workflow.
- Content projection. Content projection is the ability to pass data from parent component to child component effectively. Understanding content projection will allow you to determine the flow of data on your application.
- OnPush change detection. OnPush change detection significantly speeds up an application by ensuring that the data in the component and the display are in sync. The app’s Angular framework can listen for specific triggers and then run the change on a subtree for that component.
- Custom pipes. Custom pipes allow you to easily create filters and transform data formats to suit your needs.
5 Common Challenges That Angular Guidelines Can Address
There are certain challenges that you are likely to face while working with Angular, whether you’re a newbie or an experienced programmer. Below are some of the most common issues you will want to familiarize yourself with.
Angular App Frequently Slows Down
This is a challenge that all Angular developers face at some point while creating an app. The application will slow down to the point where the user will be forced to close it. Following Angular best practices and using onPush can prevent this from happening.
Angular App Unnecessarily Uses Cloud Space
Sometimes, your application will use up cloud space, which can violate the users’ privacy and pose a security threat to your app. This also causes the app to slow down and can hinder the development experience.
Resolves Are Overused
Resolves can slow down the performance of your app. Overusing them means it will take extra time to load your app’s display. However, they are necessary for gathering data from the API. To avoid slowing down your app with resolves, you can render some parts of the display while the data is being gathered from the API.
App Not Optimized
There are different ways that this can happen. It could be caused by too many digest loops or by not using $applyAsync. $applyAsync delays the resolution of expression until the next $digest cycle which is triggered after a zero time out.
Available Tools Not Used Correctly
You should use all of the tools available to you to ensure your app is effective. Some of these tools are Chrome Breakpoints and Chrome Timeline. If you neglect these tools, you will end up with an application that doesn’t function correctly. Make sure you have clean code and stick to the standard Angular style guide to ensure you are using the best Angular practices.
Top 10 Angular Best Practices and Guidelines
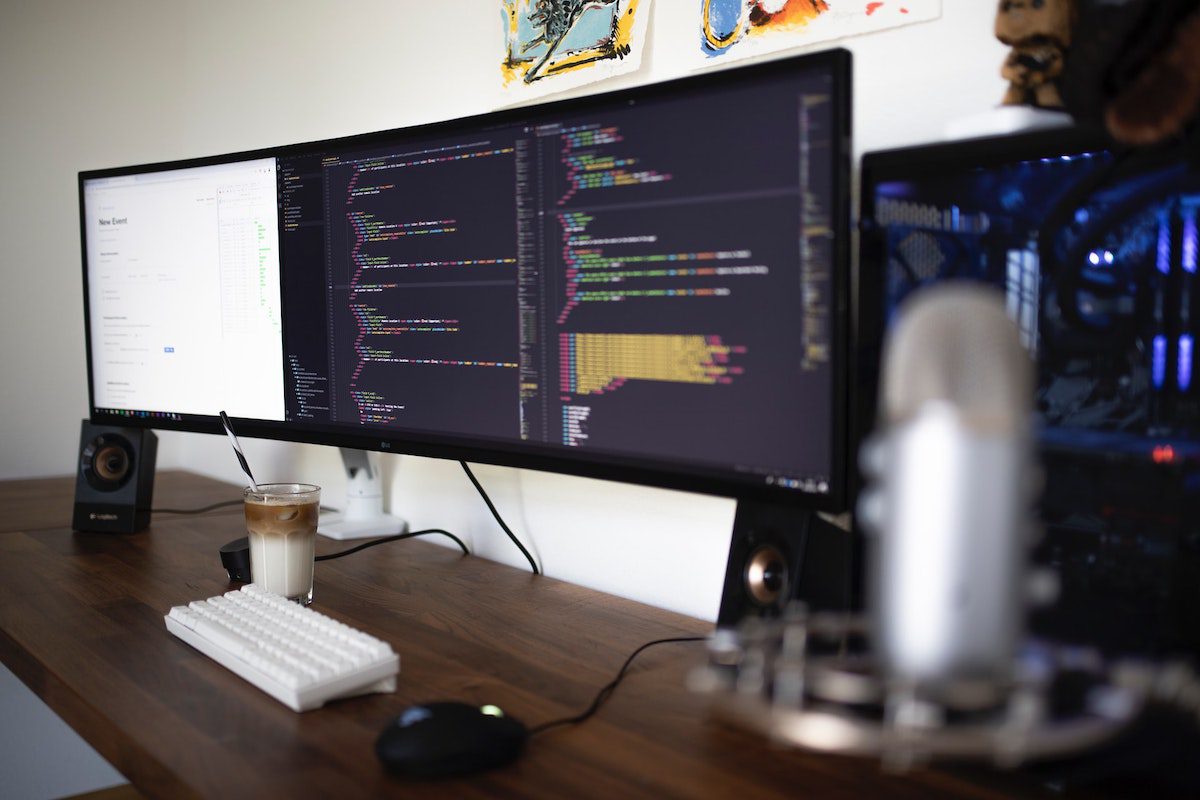
Adhering to Angular best practices will help you provide the best possible user experience by creating a secure, high-performance app. These best practices will also help you avoid preventable issues in the future. Below are some important guidelines to follow.
Use Angular CLI
Angular CLI is a command-line interface tool that allows you to easily create an application that is in line with best practices. It is used to initialize, develop, scaffold, and maintain Angular applications efficiently.
Creating files and folders manually is often considered to be a bad practice. You can use Angular CLI to generate components, directives, modules, services, and pipes. Maintaining the proper folder structure is an essential best practice when working with Angular.
Be Consistent with Angular Coding Styles
You should follow Angular coding styles to ensure that your application is up to coding standards. You will want to limit your files to 400 lines of code, include the names and properties of methods in the lowerCamelCase style, leave one empty line between application imports and modules, and create short functions with no more than 75 pieces of code.
Use trackBy Along with ngFor
It is quite common to use ngFor to loop over an array in templates. To get an even better result, you should use it with a trackBy function to get a unique identifier for each DOM item. This is done because when an array changes, Angular will re-render the entire DOM tree. TrackBY will help Angular see which element has changed and will only make changes for that element.
Break Down into Small Reusable Components
When a component becomes large, you should break it down into smaller components to reduce duplicate code. Large components are not easy to debug, manage, and test, so breaking them down will pay off later.
Use Lazy Loading
If you’re building a single-page application, the app’s initial load time can be long. Using lazy load will reduce the application boot time. With this built-in feature, only the necessary custom modules will be loaded. This will enhance productivity and keep your application clean.
Use a Scalable and Maintainable Project Structure
The default structure generated by Angular CLI is great for small apps but isn’t good for large ones, as it can be difficult to maintain and scale larger apps. To prevent this, you should use a project structure that is scalable and easy to maintain. A good structure should include a core module, shared module, feature module, and root app module for each feature of the app.
Use Interfaces
Interfaces are used to describe defined objects. You should use interfaces when creating a contract class. If they are used correctly, the class will be forced to implement functions and attributes declared in the interface.
Use Immutability
There are two types of references in JavaScript, objects and arrays. To copy an object or array for modification, you should use the es6 spread operator. To accomplish this, you will need to employ immutability. This will recreate a user object and override the status property.
Use Index.ts
Index.ts is a file in Angular that you can use to organize imports. With Index.ts, you can import multiple modules from other folders and re-export them easily, which will reduce the size of the import statement. Index.ts files provide TypeScript information about a JavaScript module.
Always Document Your Code
It is critical that you always document your code base so that you can assess its readability. You should use multiline comments to explain the tasks and parameters of the code for each variable and method. You can also make this step easier by using an extension to generate comments.
It is important to stay up to date with Angular, as the framework releases major updates every six months. By keeping up to date, you can be sure that you’re making use of the most recent features, bug fixes, and performance improvements.
How to Learn Angular Best Practices
There are different mediums that you can utilize to learn Angular best practices when you are in development mode. You can enroll in online classes that cover Angular material, join a coding bootcamp, use Angular templates, or simply read a book.
Can a Bootcamp Help You Learn Angular Best Practices?
A coding bootcamp can help you learn Angular best practices. Coding bootcamps provide students with the relevant skills needed to start a career. In this case, you’ll want to enroll in a coding bootcamp that focuses on Angular.
Bootcamps are short-term educational programs that help students learn in-demand tech skills and dynamic thinking. The curriculum is usually designed to ensure students become employable professionals, and most bootcamps include career services after graduation. If you want to enhance your coding standard and master Angular, a bootcamp is a strong option.

"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Best Courses and Training Programs to Learn Angular Best Practices
Provider | Course | Price |
---|---|---|
Codeworks | Software Engineering Bootcamp | $8,900 |
Coursera | Full Stack Web Development with Angular Specialization | Free |
Pluralsight | Angular Best Practices | Free |
Simplilearn | Angular Tutorial for Beginners | Free |
Udemy | The Modern Angular Bootcamp | $99.99 |
Should You Learn Angular Best Practices?
You should learn Angular best practices if you want to create high-quality apps. Learning the rules before you get to work on your Angular project will allow you to sidestep common issues and work with dynamic programming structures. Angular is one of the most popular frameworks, and if you want to land a job building web applications, it is a tool you will want to master.
Angular Best Practices and Guidelines FAQ
Some of the best Angular security practices include preventing cross-site scripting, blocking HTTP-related vulnerabilities, avoiding risky Angular APIs or customizing Angular files, and staying up to date on the latest Angular library.
The Angular coding standards are style vocabulary, file structure conventions, single responsibility, naming, application structure and NgModules, components, directives, services, data services, lifecycle hooks, and appendix.
Lazy loading is a technique that allows JavaScript components to be loaded simultaneously when a specific route is activated. It improves application load time by dividing the application into several bundles that can be loaded separately.
Webpack is an open-source module bundler that is used to bundle application source code into manageable chunks and load that code from a server onto a browser. Webpack searches your website for JavaScript files, then merges them into one or more large files.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.